Hi there,
Welcome back to another exploration of Salesforce development concepts. Today’s topic focuses on Apex datatypes, the building blocks of Salesforce programming. For those new to Salesforce, it’s helpful to first understand the Basics of Apex or check out How to Set Up VS Code for Salesforce Development to ensure you’re ready to follow along with the examples.
This post will delve into the various types of datatypes in Apex, explaining their purposes and demonstrating how to use them effectively in your code. Examples will be shown using VS Code, so having your environment set up in advance is essential.
Let’s begin the journey to understanding Apex datatypes and their role in creating efficient and powerful Salesforce solutions.
What Are Datatypes in Apex?
Datatypes in Apex define the kind of data a variable can hold, such as numbers, text, or dates. They are the building blocks of Salesforce code and are classified into four main types:
- Primitive Datatypes
- Collections
- SObject Datatypes
- Enums
Each datatype is crucial in structuring your data and shaping your code. Let’s go over each one with examples.
1. Primitive Datatypes
Primitive datatypes are the simplest and most frequently used datatypes. They store basic information like numbers, text, or dates.
Integer
This datatype stores whole numbers and is ideal for counts or numeric IDs.
public static void integerExample() {
Integer userCount = 150;
System.debug('User Count: ' + userCount);
}
Decimal
Decimal is used for numbers with fractional values, commonly applied in financial computations.
public static void decimalExample() {
Decimal discount = 12.5;
System.debug('Discount: ' + discount);
}
String
Strings store textual data, such as user names or system messages.
public static void stringExample() {
String greeting = 'Welcome to SFDC Insights!';
System.debug('Greeting: ' + greeting);
}
Date, Time, and DateTime
These datatypes manage dates and timestamps, often used for scheduling or logging data.
public static void dateTimeExample() {
Date today = Date.today();
DateTime now = DateTime.now();
System.debug('Today: ' + today);
System.debug('Current Time: ' + now);
}
Boolean
Boolean datatypes store true or false values, useful for conditional statements.
public static void booleanExample() {
Boolean isActive = true;
System.debug('Is Active: ' + isActive);
}
Id
The Id datatype is used for Salesforce record IDs. It is commonly used to uniquely identify records.
public static void idExample() {
Id recordId = '0012w00000J9IYr';
System.debug('Record ID: ' + recordId);
}
2. Collections
Collections allow you to group and manage multiple values in one variable. Apex provides three types of collections:
List
Lists store ordered elements and are useful when sequence matters.
public static void listExample() {
List<String> fruits = new List<String>{'Apple', 'Banana', 'Mango'};
System.debug('Fruits List: ' + fruits);
}
Set
Sets store unique elements in an unordered manner.
public static void setExample() {
Set<String> cities = new Set<String>{'London', 'Paris', 'Tokyo'};
System.debug('Cities Set: ' + cities);
}
Map
Maps store key-value pairs and are extremely handy for lookups or matching data.
public static void mapExample() {
Map<Integer, String> employeeMap = new Map<Integer, String>{
101 => 'Alice',
102 => 'Bob'
};
System.debug('Employee Map: ' + employeeMap);
}
3. SObject Datatypes
SObjects represent Salesforce records, such as Account, Contact, or custom objects. This datatype allows interaction with the Salesforce database.
public static void sObjectExample() {
Account acc = new Account(Name = 'Test Account', Phone = '1234567890');
insert acc;
System.debug('Inserted Account ID: ' + acc.Id);
}
4. Enums
Enums represent a fixed set of constants, useful for predefined values like statuses or types.
public enum Status {
Open,
InProgress,
Closed
}
public static void enumExample() {
// Assigning a value from the enum
Status currentStatus = Status.Open;
System.debug('Current Status: ' + currentStatus);
// Changing the status
currentStatus = Status.Closed;
System.debug('Updated Status: ' + currentStatus);
}
Executing the Code in VS Code
To try these examples:
- Save the code snippets into an Apex class file, e.g., DatatypeExamples.cls.
- Deploy the class to your Salesforce org using the VS Code deployment tools.
- Create a separate file and write the method like:-
DatatypeExamples.listExample();
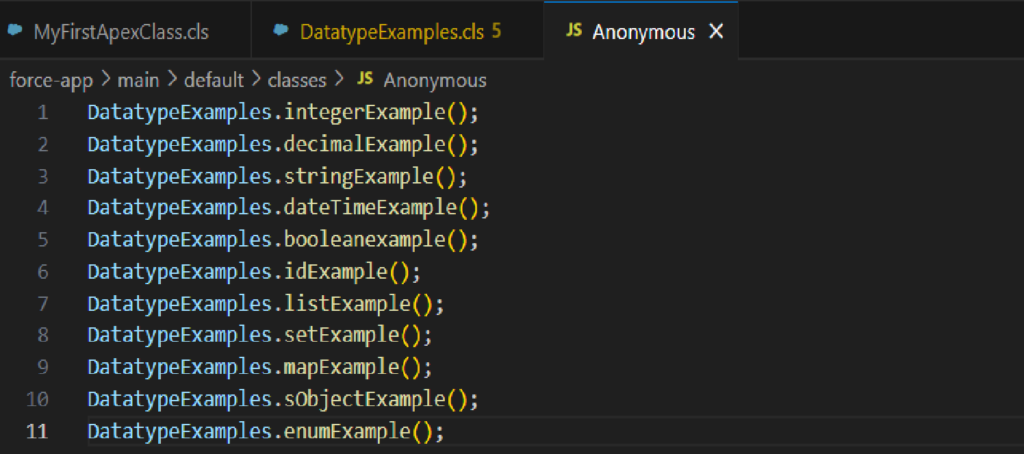
- From Command Palette, Select Execute Anonymous Apex with Editor Contents
- View the outputs in the VS Code.
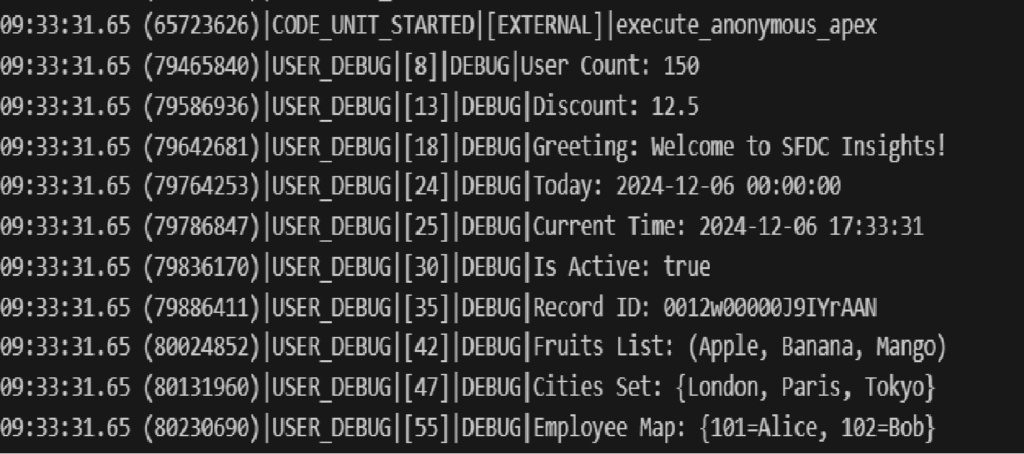

Final Thoughts
Understanding Apex datatypes is a critical step in mastering Salesforce development. With practice, you’ll gain confidence in handling data efficiently, whether you’re working with simple variables, collections, or advanced data structures like SObjects.
Stay tuned for more insightful content right here on SFDC Insights. Let’s keep learning and growing together!
#SFDCInsights #ApexDatatypes #SalesforceDevelopment