Apex is Salesforce’s programming language for automating processes and writing business logic. In this blog, we’ll walk you through how to execute Apex code directly in VS Code using the SFDX: Execute Anonymous Apex with Editor command and how to use System.debug()
to debug your code.
If you haven’t set up VS Code for Salesforce development yet, check out my guide on How to Set Up VS Code for Salesforce Development.
What is a Class in Apex?
In Apex, a class is a container for code that defines properties and methods. It is similar to classes in other programming languages like Java or C#. A class can contain variables, methods, and other logic to perform a specific function.
What is a Method in Apex?
A method is a block of code within a class that performs a specific action or task. It can take inputs (parameters) and can return a value. Methods are called to execute the logic defined within them.
Step 1: Create an Apex Class
- Press
Ctrl + Shift + P
(Windows/Linux) orCmd + Shift + P
(Mac) to open the Command Palette. - Type
SFDX: Create Apex Class
in the Command Palette and select it from the list. - When prompted, enter the class name (e.g.,
MyFirstApexClass
). - After you hit Enter, the new Apex class file will be created under the
force-app/main/default/classes
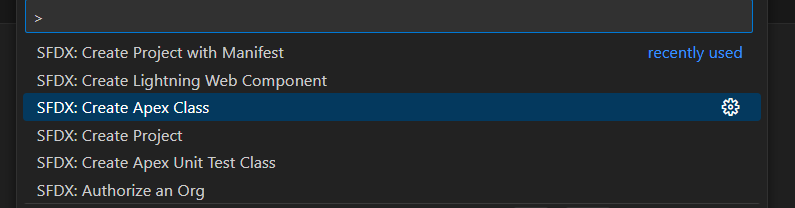
Open the newly created MyFirstApexClass.cls
file and add the following code:
public class MyFirstApexClass {
public static void greetUser(String userName) {
System.debug('Hello, ' + userName + '!');
}
}
This class defines a method greetUser
that logs a greeting to the debug log.
Step 2: Deploy the Apex Class to Your Salesforce Org
Before running the Apex code in Anonymous Apex, you need to deploy the class to your Salesforce org. Here’s how to do it easily in VS Code:
- Right-click on the MyFirstApexClass.cls file in the Explorer pane.
- Select Deploy to Org from the context menu.
- The deployment will begin, and you’ll see the status in the Output panel.
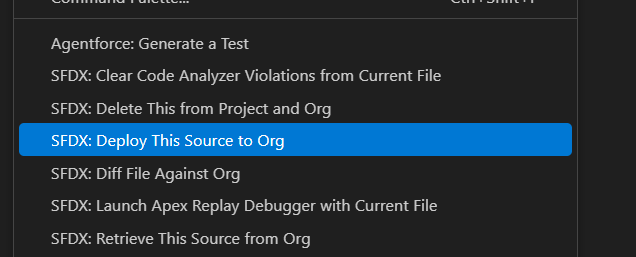
Once the deployment is successful, the class will be available on your Salesforce org, and you can proceed with testing.
Step 3: Execute Apex Code Using Ctrl + Shift + P
- Create a new file with no extension and type the following code to call the greetUser method:
MyFirstApexClass.greetUser('Himanshu');
- Press
Ctrl + Shift + P
(Windows/Linux) orCmd + Shift + P
(Mac) to open the Command Palette in VS Code. - Type and select
SFDX: Execute Anonymous Apex with Editor
. - Press Enter or select the Execute option again to run the code. This will execute the anonymous Apex code.
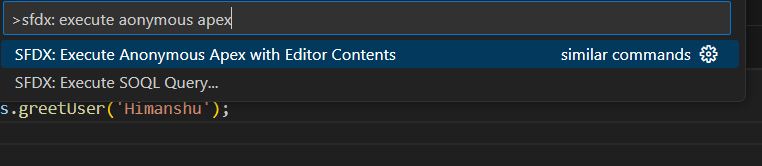
Step 4: View Debug Logs in VS Code
After executing the code, open the Output tab in VS Code.
Scroll through the output and you will find the System.debug() log, which will show the greeting message, such as:
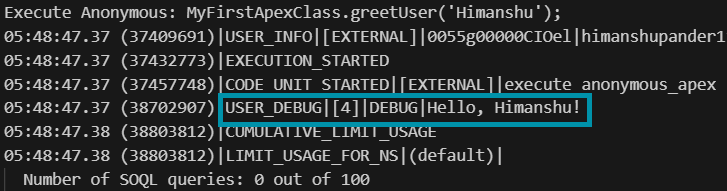
Conclusion
By following these steps, you’ve learned how to:
- Create an Apex class and method in VS Code.
- Easily deploy the class to your Salesforce org using Deploy to Org.
- Execute Apex code using SFDX: Execute Anonymous Apex with Editor.
- View System.debug() output in the Output Panel.
This approach makes it easy to write, test, and deploy Apex code directly from VS Code.
Call to Action
If you found this blog helpful, feel free to share it or leave a comment. For more Salesforce tips and tutorials, check out SFDC Insights!
Gгeate post. Keеp posting such kind of information on your blog.
Im really impressed by it.
Hellⲟ theгe, You have performed a great job.
I’ll definitely digg it and for my part sugցeѕt to my friends.
I am sure they’ll be benefited from this website.
Thank you! I’m glad that this post was helpful for you. Stay Tuned for more such blogs!
Pingback: What are DataTypes in Apex – SFDC INSIGHTS